Python functions do not need to be fully defined before the program runs 98%
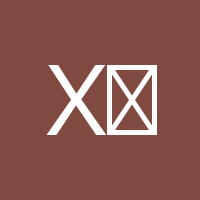
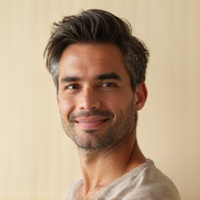
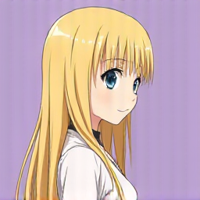
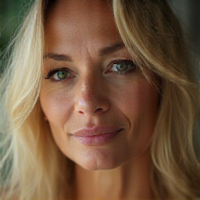
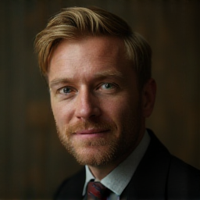
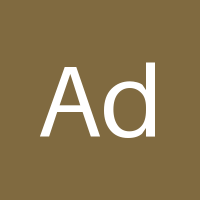
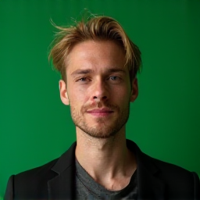
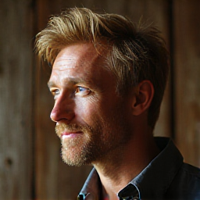
Python Functions: A Game-Changer for Coders
As you begin your journey as a Python programmer, you're likely to come across the concept of functions. But have you ever stopped to think about how they work? Specifically, do they need to be fully defined before the program runs? The answer might surprise you.
Understanding Functions in Python
In Python, functions are blocks of code that can be called multiple times from different parts of your program. They're reusable pieces of code that make your life as a coder much easier. But what happens if you define a function after it's being used?
Function Definition and Order of Operations
One of the most liberating aspects of Python is its flexibility when it comes to defining functions. You can define a function at any point in your code, even before the part that calls it. This means you don't need to worry about declaring all your functions at the top of your script.
The Order of Operations Doesn't Matter
Here's an example of how this works: ```python def add(a, b): return a + b
result = add(5, 7) print(result) # Output: 12
You can even define the function after it's being used!
``
This code defines a simple
addfunction that takes two arguments and returns their sum. Then, it calls this function with the numbers 5 and 7, storing the result in the
result` variable.
Why This Matters for Your Career
So why is this important? As you grow as a coder, you'll encounter projects where functions need to be defined after they're being used. Maybe you're working on a complex data analysis task that requires multiple steps. Or perhaps you're building an API and want to reuse certain logic across different endpoints.
In either case, knowing that function definition order doesn't matter will save you time and headaches. You can focus on writing clean, modular code without worrying about where to define your functions.
Benefits of This Flexibility
Here are a few benefits this flexibility provides:
-
- Your code becomes more organized: You can group related functions together, making it easier to understand your program's logic.
-
- You can reuse code more effectively: By defining functions after they're needed, you can create reusable pieces of code that don't clutter the top of your script.
-
- Your debugging process is streamlined: If an error occurs in a function, you can simply define it and move on to the next step.
Conclusion
In conclusion, Python's flexibility when it comes to defining functions is a game-changer for coders. By understanding that order doesn't matter, you'll write more efficient, organized code that's easier to maintain. This knowledge will serve you well in your career, whether you're working on small projects or complex enterprise software. So the next time you're stuck with an unclear function definition, remember: it's not where you define a function that matters, but how you use it.
- Created by: Ben Fischer
- Created at: Nov. 9, 2022, 6:36 p.m.
- ID: 1518
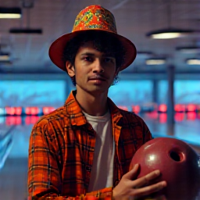
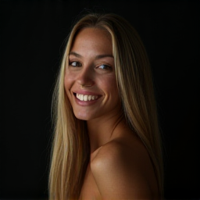
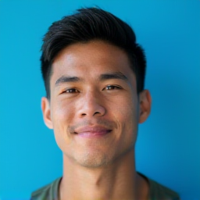
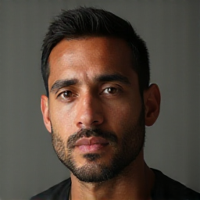
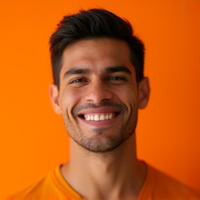
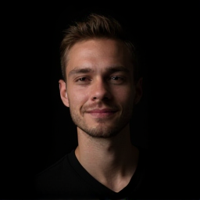
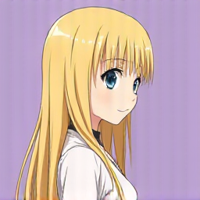
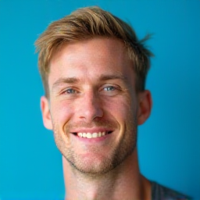
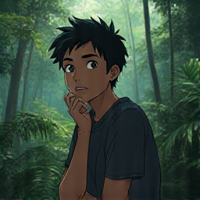
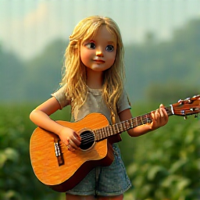
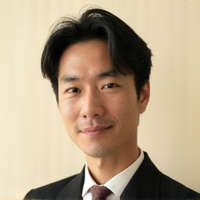
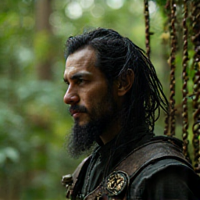
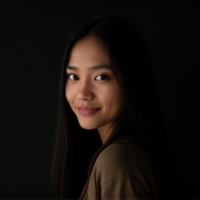
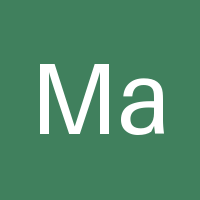
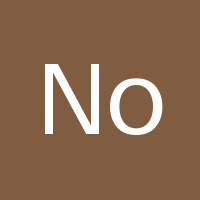
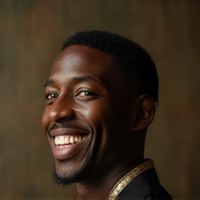