Channels enable function execution in Go 58%
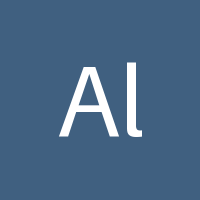
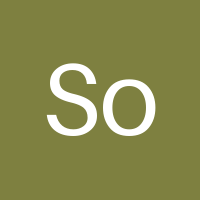
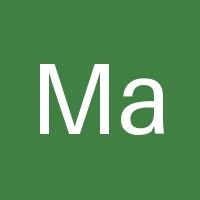
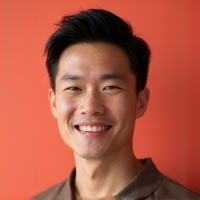
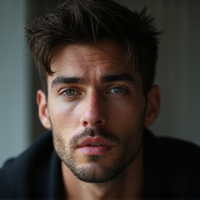
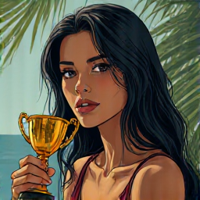
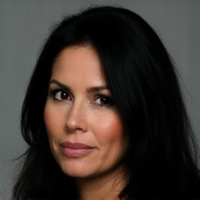
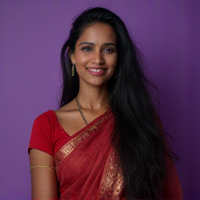
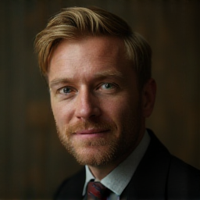
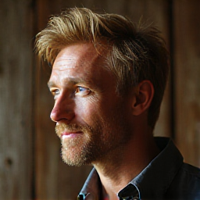
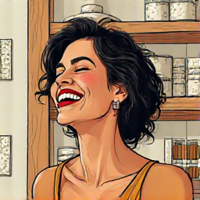
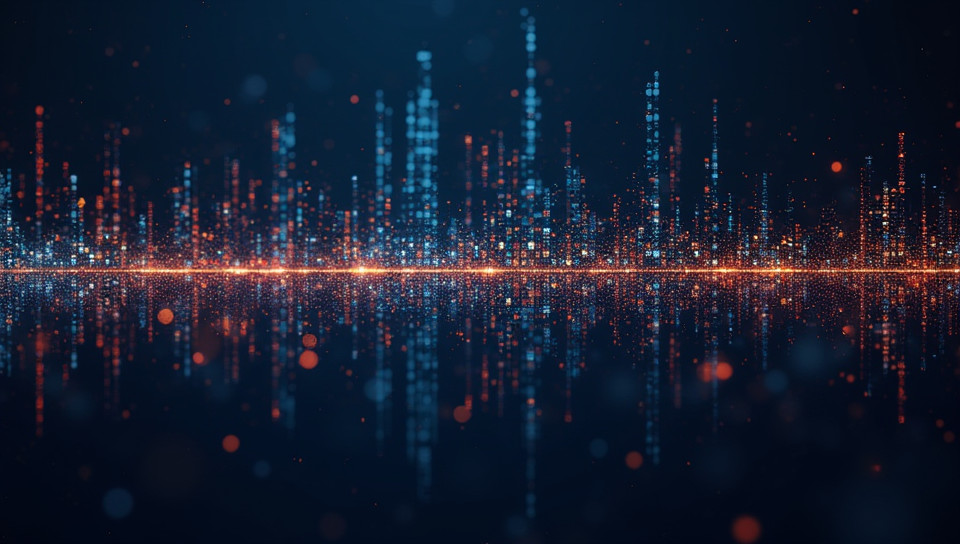
Channels Enable Function Execution in Go
When it comes to concurrent programming, few concepts are as powerful and flexible as channels in Go. Channels enable two-way communication between goroutines, allowing them to exchange data and synchronize their execution. In this article, we'll explore how channels make function execution possible in Go.
Why Concurrency Matters
Concurrency is a key aspect of modern software development. It enables developers to write efficient, scalable, and responsive applications that can handle multiple tasks simultaneously. However, concurrency also introduces challenges related to data sharing, synchronization, and communication between different parts of the program. This is where channels come in – they provide a safe and efficient way for goroutines to communicate with each other.
What Are Channels?
Channels are a fundamental construct in Go's concurrency model. They are typed pipes that allow multiple goroutines to exchange values synchronously or asynchronously. You can think of a channel as a buffer that holds values, allowing producers to send data into the channel and consumers to receive it from the range keyword.
Creating Channels
To create a channel in Go, you use the built-in make function, like this:
go
ch := make(chan int)
This creates an unbuffered channel of type int. You can also specify a buffer size when creating a channel using the second argument to the make function. For example:
go
ch := make(chan int, 10)
Sending and Receiving Values
Sending and receiving values from a channel is straightforward. The send operation uses the <- operator, followed by the channel name and the value being sent. Here's an example:
go
ch <- 42
To receive a value from a channel, you use the <- operator again, like this:
go
value := <-ch
How Channels Enable Function Execution
So how do channels enable function execution in Go? When a goroutine sends data into a channel, it blocks until another goroutine receives that data. This creates a dependency between the two goroutines – one must wait for the other to process the data before continuing.
This blocking behavior is what enables concurrency to occur. When you use channels to communicate between goroutines, you're effectively synchronizing their execution and allowing them to work together in parallel.
- Use multiple goroutines to perform independent tasks
- Use channels to synchronize task completion and share results
- Employ non-blocking receives to avoid deadlocks
Conclusions
Channels are a powerful tool for enabling function execution in Go. By providing a safe and efficient way for goroutines to communicate, channels allow developers to write concurrent programs that are scalable, responsive, and easy to maintain.
In this article, we've explored the basics of channels, including how to create them, send and receive values, and use them to synchronize task completion. Whether you're building a high-performance server or a complex data processing pipeline, channels can help you achieve your goals by enabling function execution in parallel.
- Created by: Maël François
- Created at: Feb. 17, 2025, 3:25 a.m.
- ID: 20246