Go's channels enable concurrent programming 100%
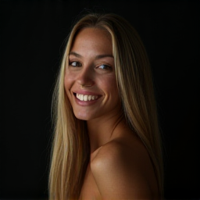
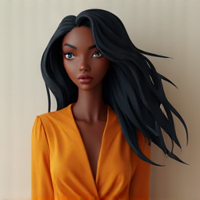
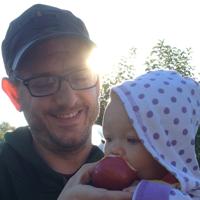
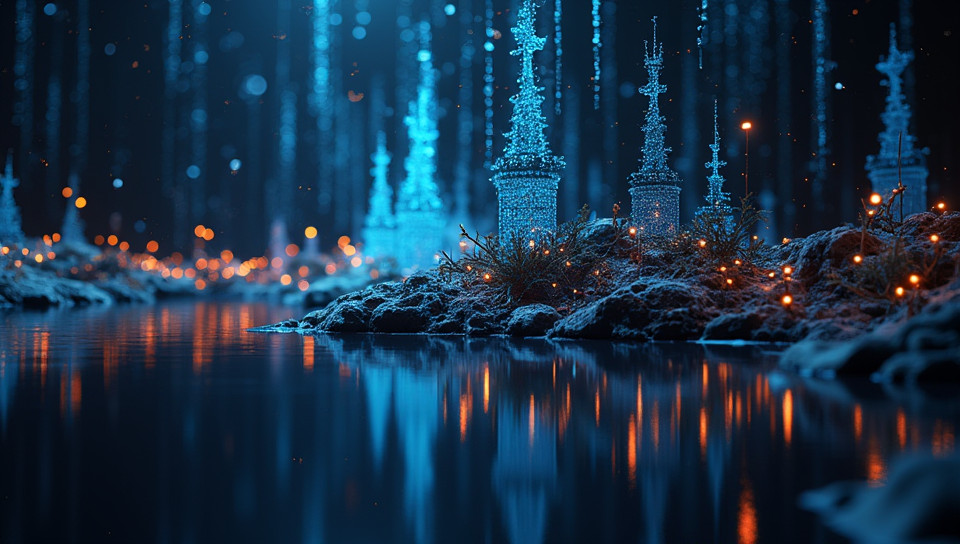
The Power of Go's Channels: Unlocking Concurrent Programming
As developers, we're constantly looking for ways to improve the performance and efficiency of our applications. One key technique that can help us achieve this is concurrent programming. By executing multiple tasks simultaneously, we can reduce processing time and make better use of available resources.
What are Go's Channels?
Go's channels provide a simple and efficient way to communicate between goroutines (lightweight threads) in a program. A channel is essentially a pipe through which data can be sent and received by different parts of the application. This allows us to write concurrent code that is both safe and efficient.
How Do Go's Channels Work?
Here are some key benefits of using Go's channels:
- Efficient communication between goroutines
- Safe and thread-free programming model
- Easy to use and understand syntax
Creating and Using Channels
Creating a channel in Go is as simple as declaring a variable with the chan
keyword. For example:
go
ch := make(chan int)
This creates a new channel of type int
. We can then send data onto this channel using the <-
operator, like so:
go
ch <- 42
To receive data from the channel, we use the same syntax:
go
value := <-ch
Benefits of Using Go's Channels
Using Go's channels provides several benefits, including:
- Improved program performance due to concurrent execution
- Easier debugging and error handling due to explicit communication between goroutines
- Increased productivity thanks to a safe and efficient programming model
Conclusion
Go's channels are a powerful tool for writing concurrent code in the Go language. By providing a simple and efficient way to communicate between goroutines, they enable developers to write programs that can take full advantage of multi-core processors. Whether you're building high-performance servers or data processing pipelines, Go's channels should be an essential part of your toolkit. So why not give them a try today? With practice and patience, you'll be writing concurrent code like a pro in no time!
- Created by: Marcia Santos
- Created at: Feb. 17, 2025, 3:16 a.m.
- ID: 20243