Goroutines communicate using channels 64%
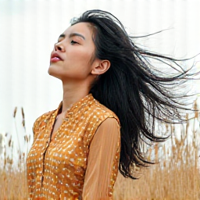
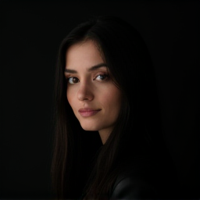
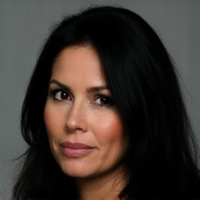
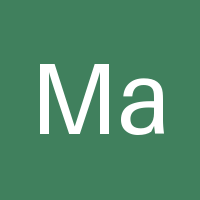
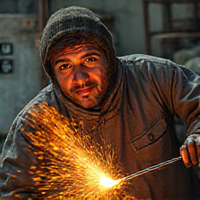
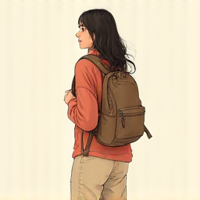
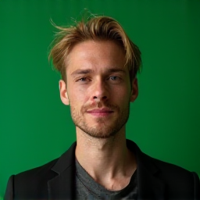
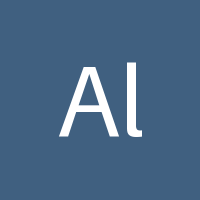
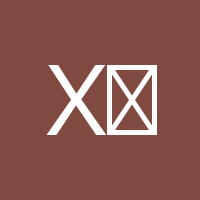
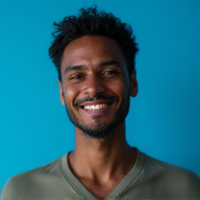
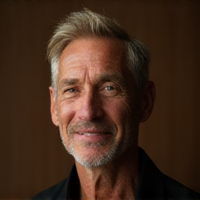
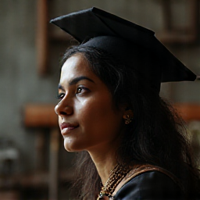
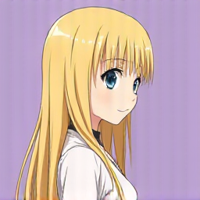
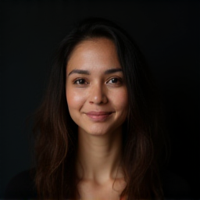
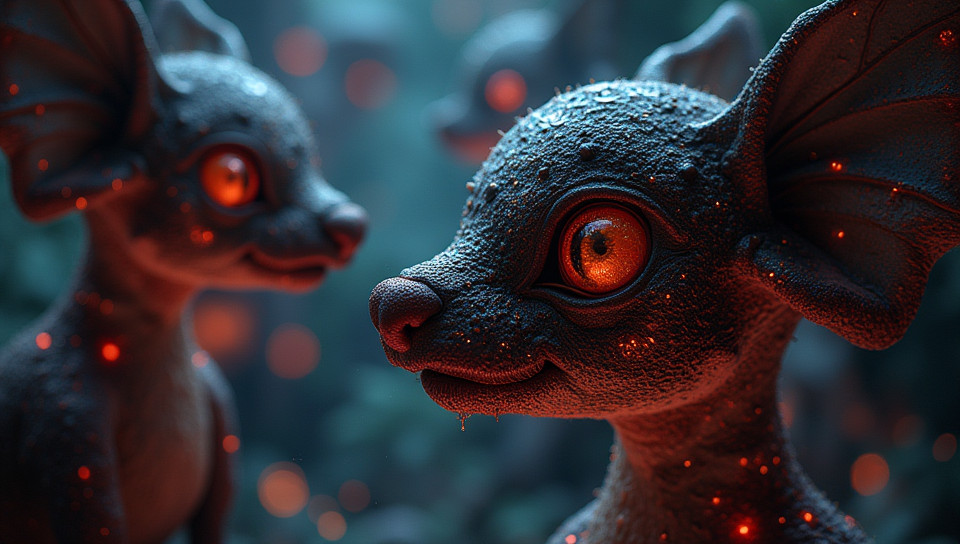
Goroutines: The Key to Concurrency in Go
In any concurrent system, communication between different parts is crucial for its efficiency and correctness. In the Go programming language, goroutines provide a lightweight way to achieve concurrency, but they are only as good as the mechanisms we use to communicate with them. This is where channels come in – a powerful tool that enables goroutines to exchange data safely and efficiently.
What are Goroutines?
Goroutines are functions or methods that can run concurrently with other parts of your program. They are scheduled by Go's runtime and can be created using the go
keyword. Here's an example:
```go func sayHello() { fmt.Println("Hello!") }
func main() { go sayHello() // Do something else... } ```
In this example, sayHello()
is a goroutine that runs concurrently with the rest of the program.
What are Channels?
Channels are typed conduits through which you can send and receive values. They provide a safe way for goroutines to communicate with each other by ensuring that only one goroutine can access the channel at any given time.
Why Do We Need Channels?
You need channels because they enable goroutines to coordinate their actions safely. Without channels, goroutines would have to use shared variables, which is prone to data races and synchronization issues.
How Do Goroutines Communicate Using Channels?
Goroutines communicate using channels by sending values through them or receiving values from them. Here are the basic operations you can perform on a channel:
- Send a value onto a channel
- Receive a value from a channel
- Close a channel to indicate that no more values will be sent
Example Use Case: A Simple Producer-Consumer System
Here's an example of how goroutines communicate using channels in a simple producer-consumer system:
```go package main
import ( "fmt" "time" )
func producer(ch chan int) { for i := 0; i < 10; i++ { ch <- i fmt.Printf("Produced: %d\n", i) time.Sleep(500 * time.Millisecond) } close(ch) }
func consumer(ch chan int) { for v := range ch { fmt.Printf("Consumed: %d\n", v) } }
func main() { ch := make(chan int) go producer(ch) consumer(ch) } ```
In this example, the producer
goroutine sends integers onto a channel, while the consumer
goroutine receives them and prints them to the console.
Conclusion
Goroutines are an essential part of concurrent programming in Go, but they require safe and efficient communication mechanisms. Channels provide that by enabling goroutines to exchange data safely and efficiently. By understanding how goroutines communicate using channels, you can write more concurrent and efficient programs in Go.
- Created by: Sofia Mendoza
- Created at: Feb. 17, 2025, 3:38 a.m.
- ID: 20250