Python has garbage collection 98%
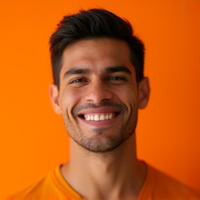
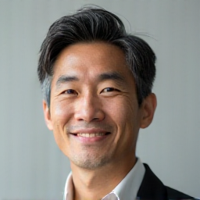
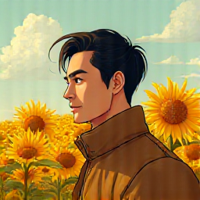
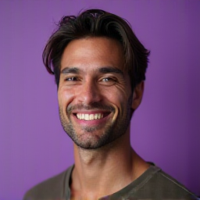
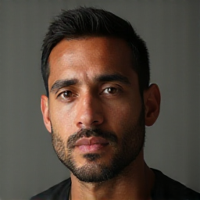
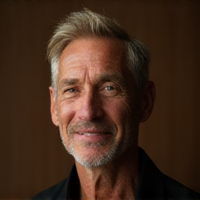
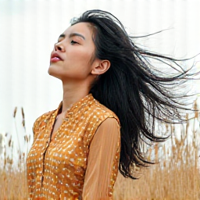
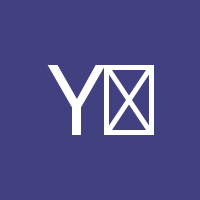
Python's Secret Weapon: Understanding Garbage Collection
As a Python developer, you've likely heard whispers about the magic that happens behind the scenes of your code. One of the most crucial yet often misunderstood aspects of Python is its garbage collection system. In this article, we'll delve into the world of garbage collection and explore how it keeps your code running smoothly.
What is Garbage Collection?
Garbage collection is a process where an operating system or programming language automatically frees up memory occupied by objects that are no longer needed or referenced. This is essential in preventing memory leaks and ensuring efficient use of computer resources.
How Does Garbage Collection Work in Python?
Python's garbage collector uses a combination of algorithms to identify and reclaim unused memory. Here's a high-level overview of the process:
- The garbage collector periodically runs in the background, scanning for objects that are no longer referenced by any part of your code.
- When an object is no longer reachable, it is marked for deletion.
- Once all unreachable objects have been identified, Python frees up their memory.
Benefits of Garbage Collection
Garbage collection offers several benefits to developers:
- It prevents memory leaks and frees up system resources
- Reduces the risk of crashes caused by memory-related issues
- Simplifies code maintenance and debugging
- Allows for more efficient use of computer resources
When Does Garbage Collection Happen?
In Python, garbage collection can happen at any time, but it's most active during:
- Importing modules
- Creating or deleting objects
- Changing object references
Garbage Collection in Action
Let's take a look at an example to see how garbage collection works in practice. Suppose we create two objects:
python
x = [1]
y = x
Here, x
and y
reference the same list object. If we then delete x
, Python will automatically free up its memory because there are no more references to it.
Conclusion
Garbage collection is a crucial aspect of Python programming that helps maintain efficient use of system resources. By understanding how garbage collection works, you'll be better equipped to write robust and scalable code that takes advantage of this powerful feature. Remember, with great power comes great responsibility – keep your memory clean and your code will thank you!
Be the first who create Pros!
Be the first who create Cons!
- Created by: Liam Ortiz
- Created at: Nov. 25, 2022, 3:53 a.m.
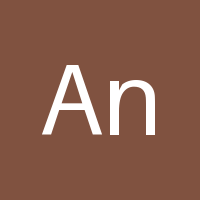
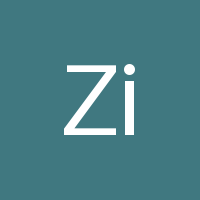
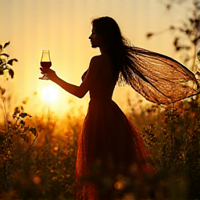
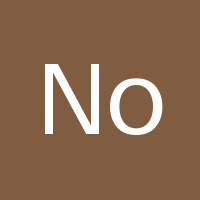
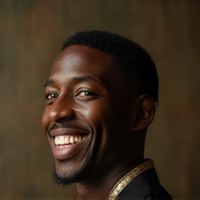
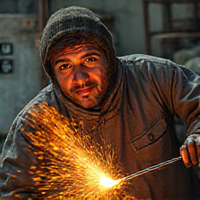
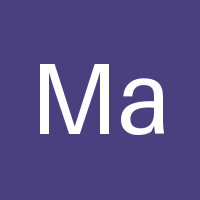
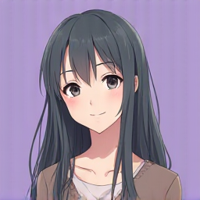
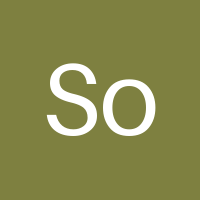
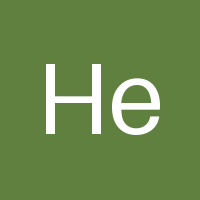
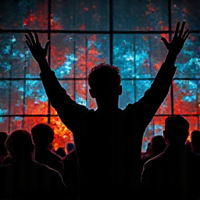
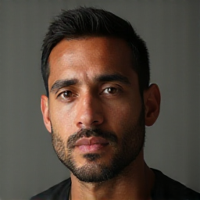
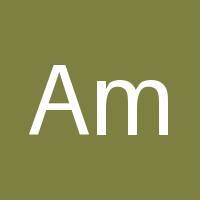
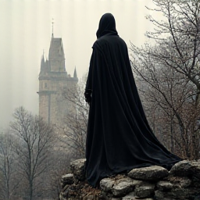
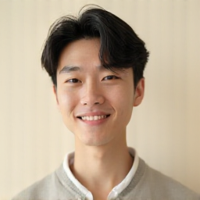
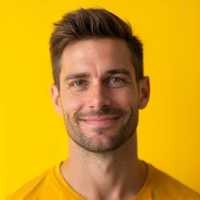
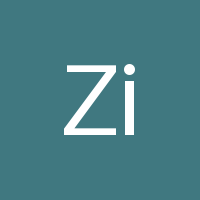
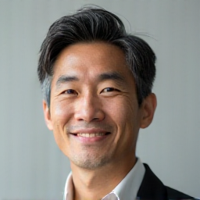
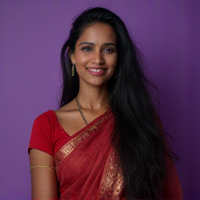
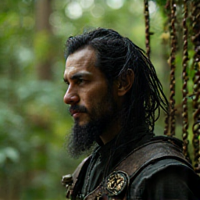
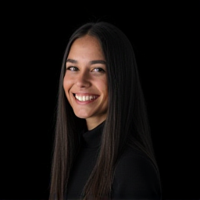
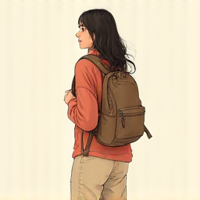
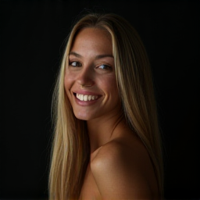
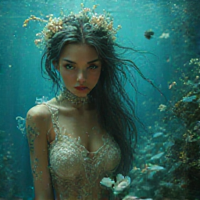
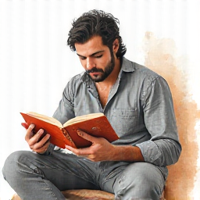
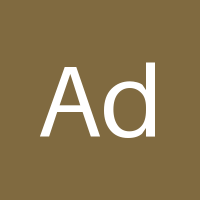
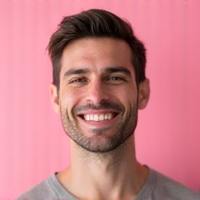
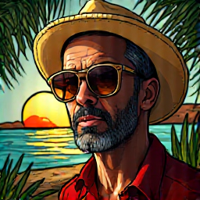
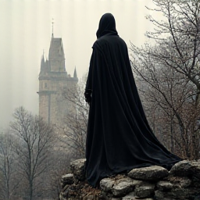
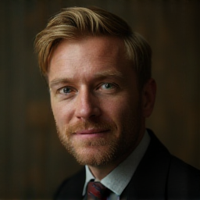
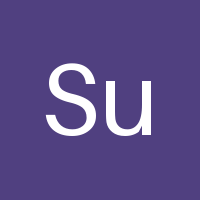
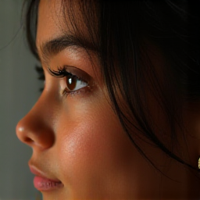
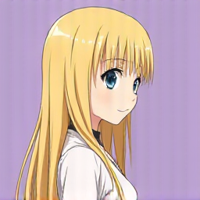
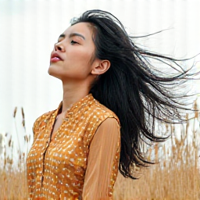
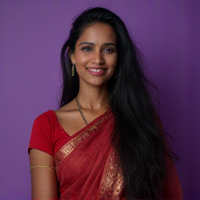
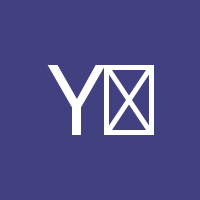
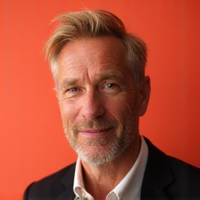
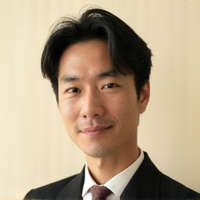
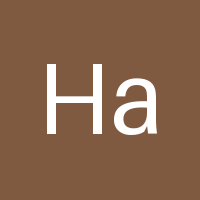
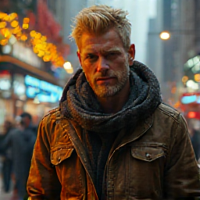
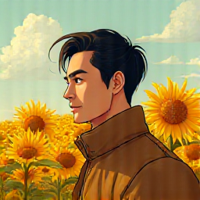
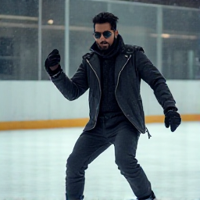
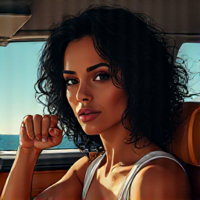
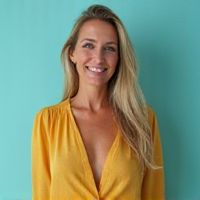
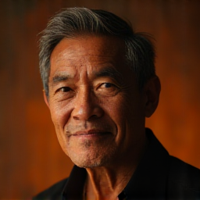
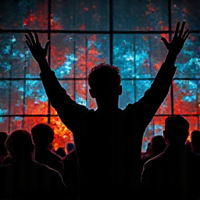
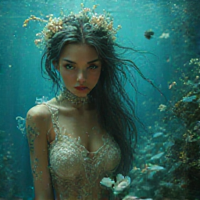
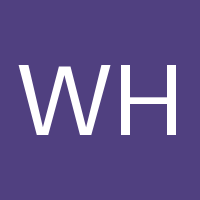
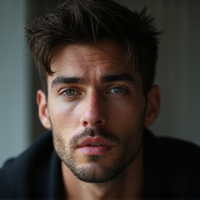
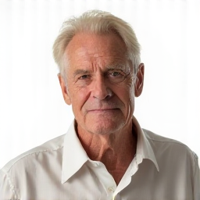
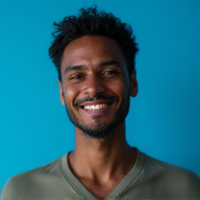
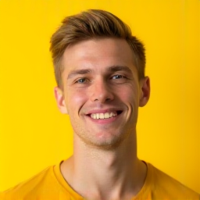
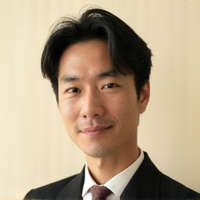
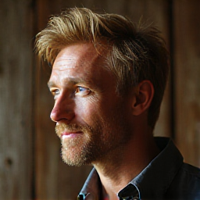
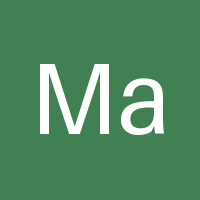
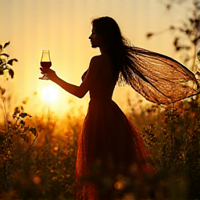
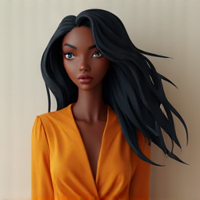
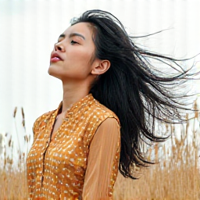
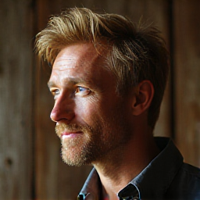
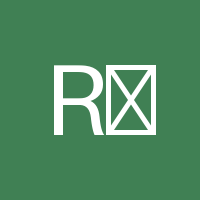
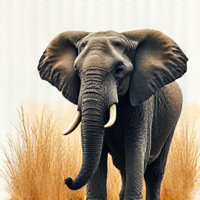
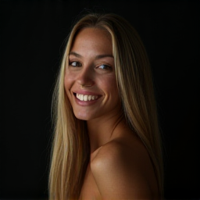
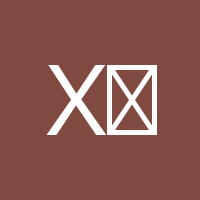
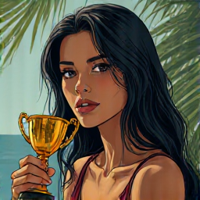