Python has large memory consumption 100%
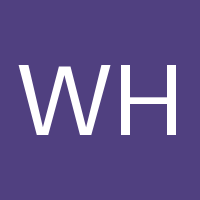
The Memory Monster: Taming Python's Large Memory Consumption
As a developer, you've likely encountered the frustration of dealing with memory-intensive applications written in Python. Whether it's a data science project, a web scraper, or a machine learning model, Python's large memory consumption can bring even the most powerful machines to their knees. In this article, we'll delve into the reasons behind Python's memory usage and explore strategies for optimizing your code to run smoothly.
Why Does Python Have Large Memory Consumption?
Python's dynamic typing system and high-level abstractions make it an ideal language for rapid development and prototyping. However, these same features can lead to inefficiencies in terms of memory usage. Here are some key factors that contribute to Python's large memory consumption:
- Object overhead: In Python, every object has a header that stores metadata such as its type, reference count, and other attributes.
- Reference counting: When you assign an object to a variable, it creates a new reference to the object. This can lead to multiple references to the same object, resulting in increased memory usage.
- Garbage collection: Python's automatic garbage collector periodically sweeps through the heap, identifying and deleting objects that are no longer referenced. While this process is efficient, it can introduce pauses in your application.
Understanding Memory Usage Patterns
To tackle Python's large memory consumption, it's essential to understand how your code uses memory. Here are some key concepts to keep in mind:
- Memory allocation: When you create an object or assign a value to a variable, memory is allocated to store that data.
- Memory deallocation: When an object goes out of scope or is deleted, the memory it occupied should be released.
Strategies for Optimizing Memory Usage
Now that we've covered the reasons behind Python's large memory consumption and the importance of understanding memory usage patterns, let's explore some strategies for optimizing your code:
- Use efficient data structures: Choose data structures like NumPy arrays or Pandas DataFrames, which are optimized for performance and memory efficiency.
- Minimize object creation: Reduce the number of objects created by reusing existing ones or using container classes like lists or dictionaries.
- Avoid unnecessary copies: Use immutability and memoization to avoid creating unnecessary copies of data.
- Profile your code: Use tools like
memory_profiler
orline_profiler
to identify memory-intensive sections of your code.
Conclusion
Python's large memory consumption is a common challenge many developers face. By understanding the reasons behind this phenomenon and applying strategies for optimizing memory usage, you can write more efficient and scalable applications. Remember to choose efficient data structures, minimize object creation, avoid unnecessary copies, and profile your code to identify areas for improvement. With these techniques in mind, you'll be well on your way to taming Python's memory monster and unlocking the full potential of this powerful language.
Be the first who create Pros!
Be the first who create Cons!
- Created by: whysage
- Created at: Nov. 25, 2022, 4:02 a.m.
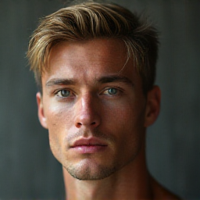
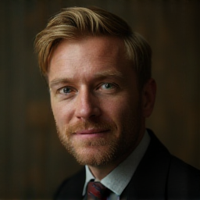
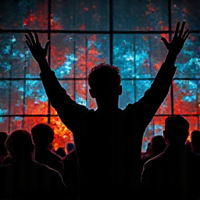
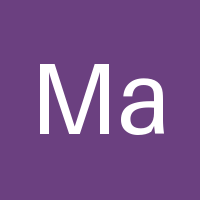
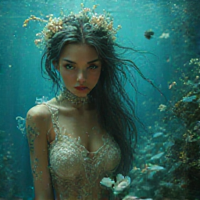
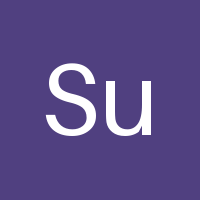
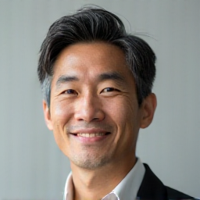
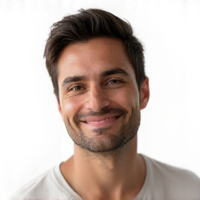
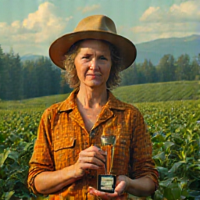
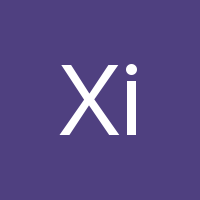
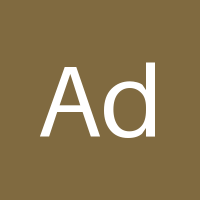
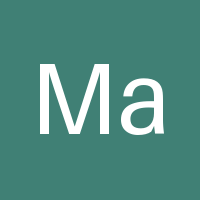
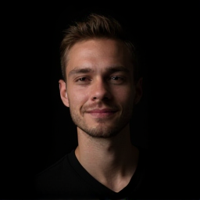
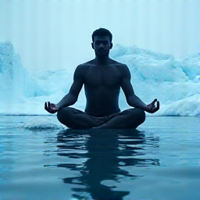
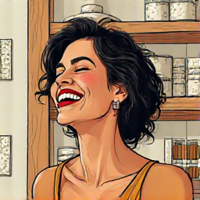
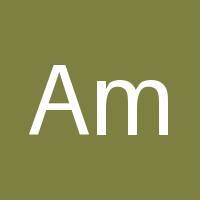
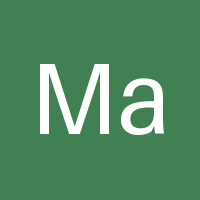
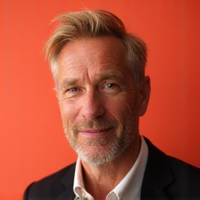
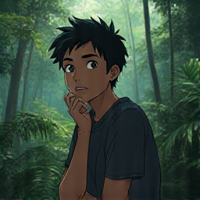
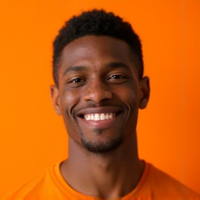
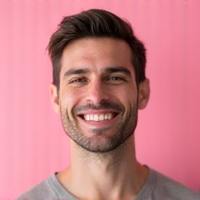
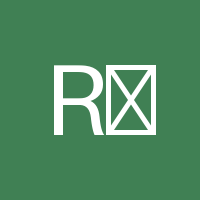
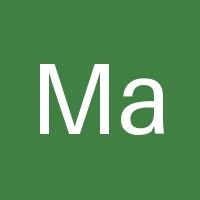
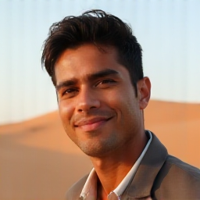
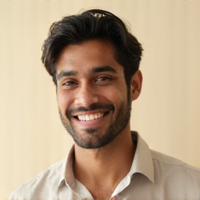
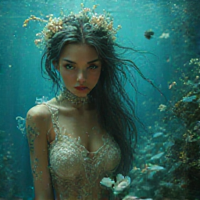
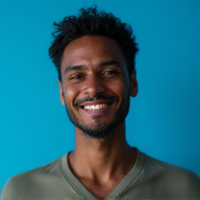
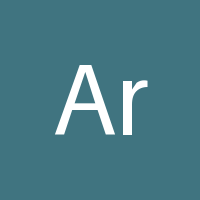
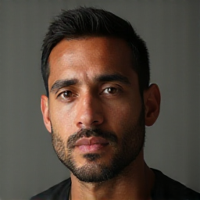
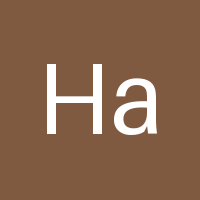
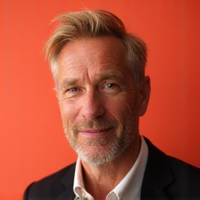
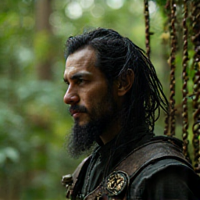
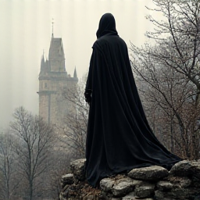
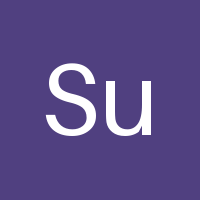
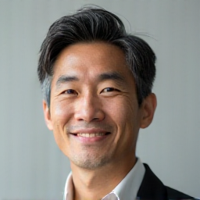
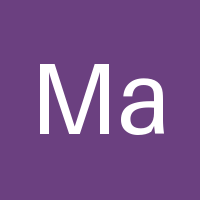
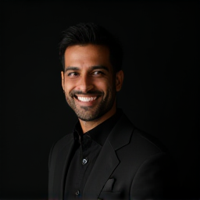
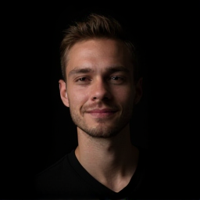
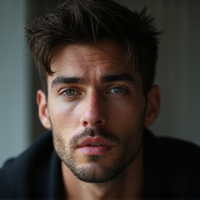
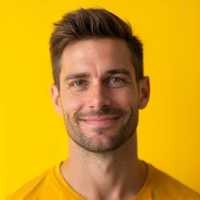
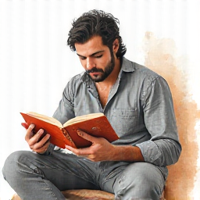
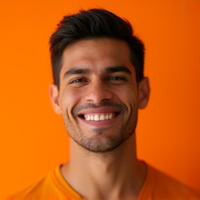
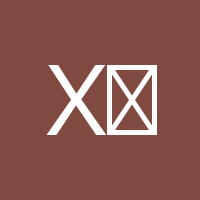
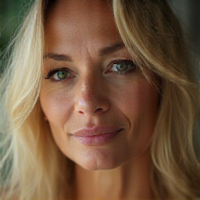
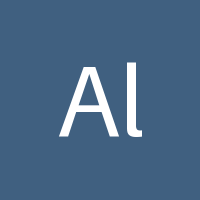
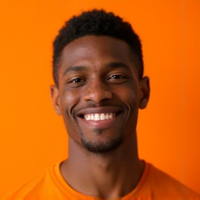
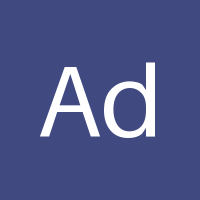
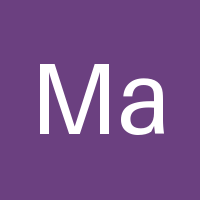
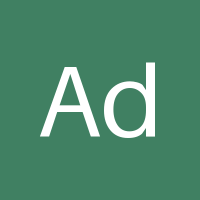
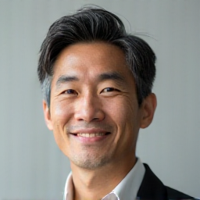
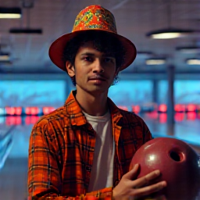
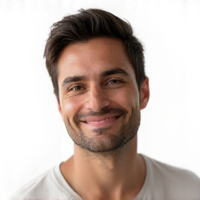
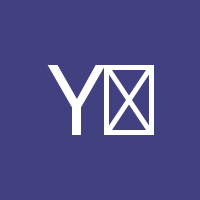
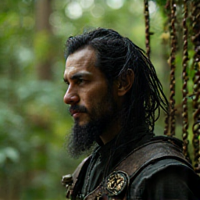
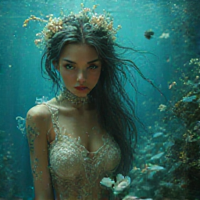
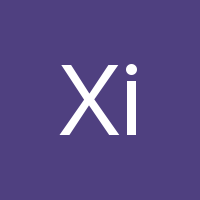
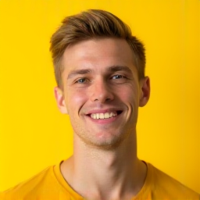
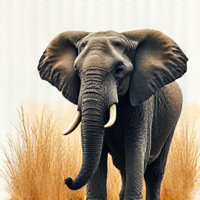
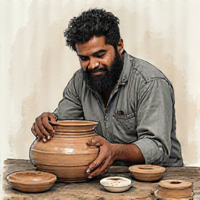
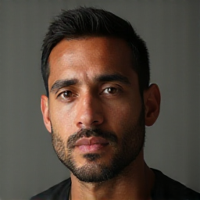
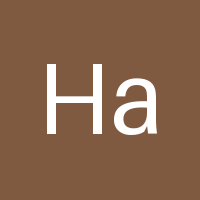
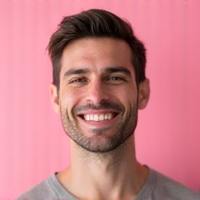
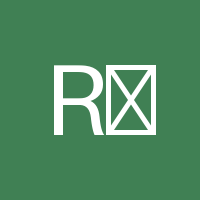
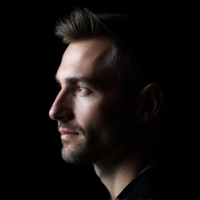
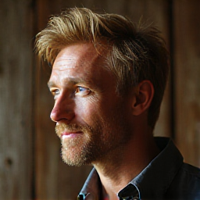
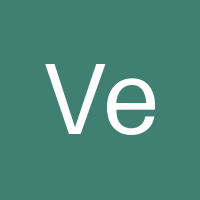
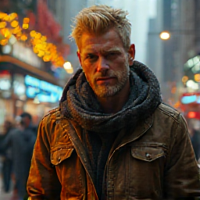
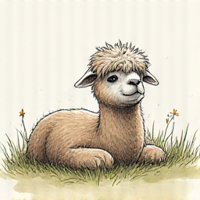
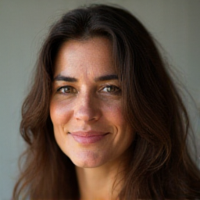
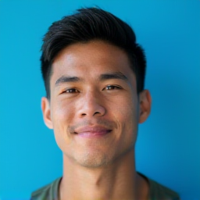
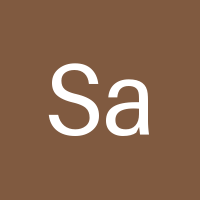
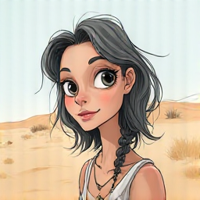