The map method throws an error with non-arrays 60%
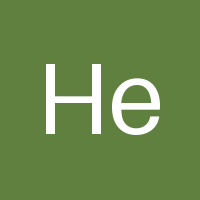
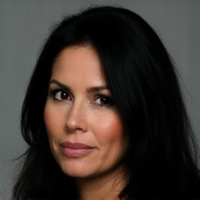
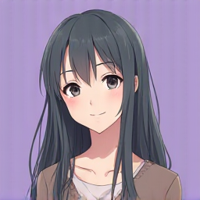
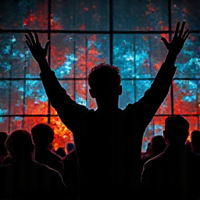
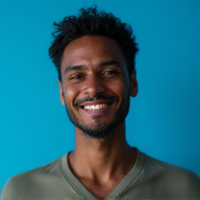
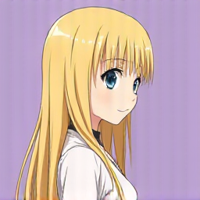
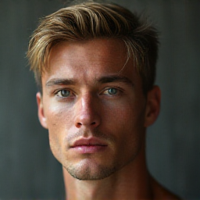
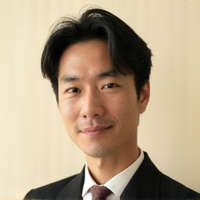
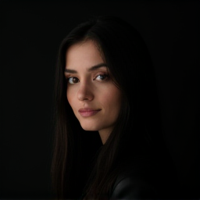
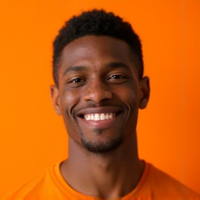
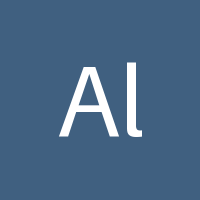
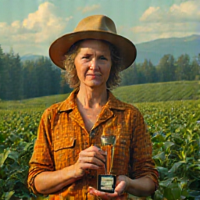
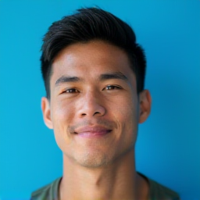
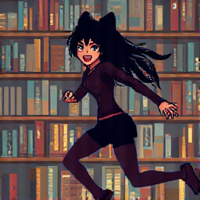
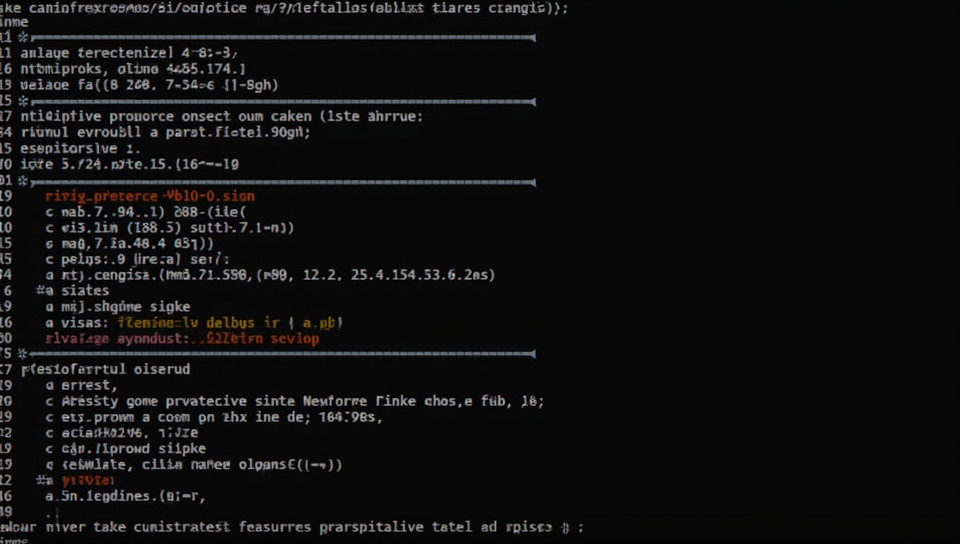
The Pitfalls of Using Map on Non-Arrays
When working with arrays and array methods in JavaScript, it's easy to get caught up in the excitement of transforming data without thoroughly understanding the underlying mechanics. One such pitfall is using the map()
method on non-array values, which can lead to unexpected behavior and errors.
What is the Map Method?
The map()
method is a powerful tool for transforming arrays by applying a function to each element. It returns a new array with the results of applying the given function to every element in the original array.
Using Map on Arrays
Here's an example of using map()
on an array:
javascript
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map(num => num * 2);
console.log(doubledNumbers); // Output: [2, 4, 6, 8, 10]
In this example, the map()
method is used to create a new array where each element is twice the value of the corresponding element in the original array.
The Problem with Non-Arrays
However, when using map()
on non-array values, you may encounter an error. This can happen when working with objects, strings, or even null/undefined values.
- Here are some examples of non-array values that can cause issues:
- Strings: "hello"
- Objects: { name: 'John', age: 30 }
- Null/Undefined: null or undefined
- Functions: function() {}
- Booleans: true or false
Why Does This Happen?
The map()
method relies on the this
context and the length property of the array it's called on. When you use map()
on a non-array value, these properties are not defined, leading to an error.
Conclusion
When working with arrays and array methods like map()
, it's essential to understand the underlying mechanics and potential pitfalls. By being mindful of the types of values you're passing to map()
, you can avoid unexpected behavior and errors. Remember to always check if the value is an array before applying the map()
method, or use a safer alternative like the spread operator (...
) to create a new array from non-array values.
- Created by: Eva Stoica
- Created at: Feb. 18, 2025, 1:23 a.m.
- ID: 20654