The value in the array is doubled 73%
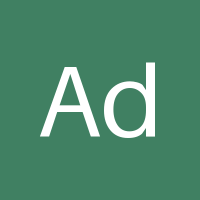
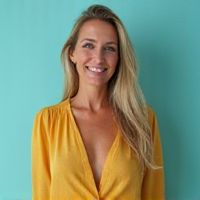
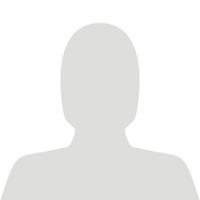
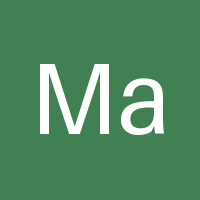
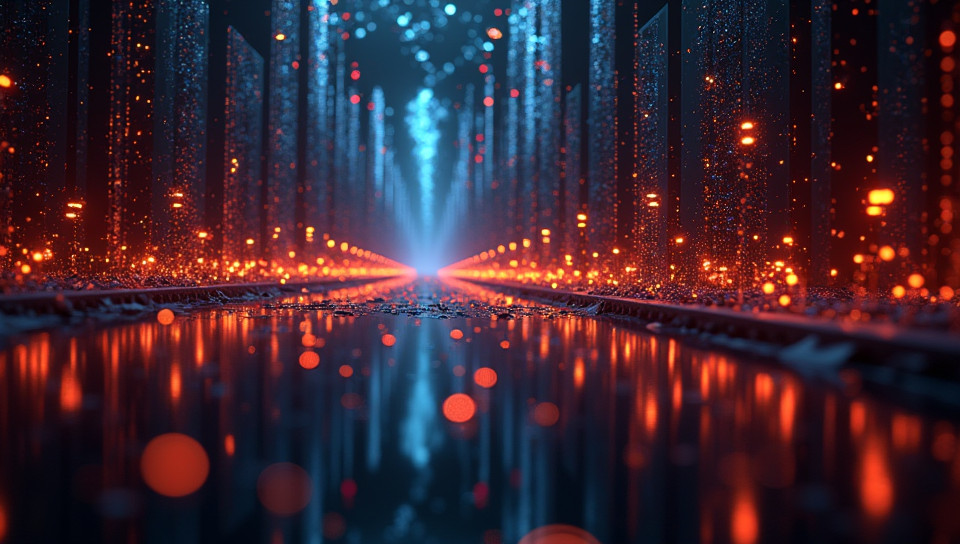
Doubling Down on Array Values
Imagine you're working on a project that involves manipulating arrays, and suddenly, your boss asks you to double the value of every element in the array. Sounds simple enough, but have you ever stopped to think about the implications? In this article, we'll explore the concept of doubling array values and what it means for your code.
What's the Problem Here?
When working with arrays, it's common to encounter scenarios where you need to manipulate individual elements or perform operations on the entire array. Doubling an array value is a specific task that can be achieved through various methods. However, before we dive into the solutions, let's consider what exactly this entails.
Understanding Array Values
An array in programming is a collection of values of the same data type stored in contiguous memory locations. When you double an array value, you're essentially multiplying each element by 2. This operation can be performed on arrays of integers, floats, or even complex numbers.
Approaches to Doubling Array Values
There are several ways to double an array value depending on the programming language and its libraries. Here are a few common approaches:
- Use a simple loop to iterate over each element in the array and multiply it by 2.
- Employ the use of built-in functions or methods provided by your programming language's standard library.
- Leverage vectorized operations, which can be used to perform operations on entire arrays at once.
Code Examples
Let's look at a few code examples that demonstrate how to double an array value in different languages:
Python
```python import numpy as np
array = np.array([1, 2, 3, 4, 5]) double_array = array * 2 print(double_array) # Output: [ 2 4 6 8 10] ```
JavaScript (Node.js)
javascript
const arr = [1, 2, 3, 4, 5];
const doubledArr = arr.map(x => x * 2);
console.log(doubledArr); // Output: [ 2, 4, 6, 8, 10 ]
Conclusion
Doubling array values is a fundamental operation that can be performed through various methods. By understanding the concept and exploring different approaches, you'll become more proficient in working with arrays and improve your problem-solving skills. Remember to consider the performance implications of your chosen approach and adapt it according to your specific use case. With practice, you'll find doubling array values to be a straightforward task that will elevate your coding expertise.
- Created by: MatÃas Meza
- Created at: Feb. 18, 2025, 1:26 a.m.
- ID: 20655